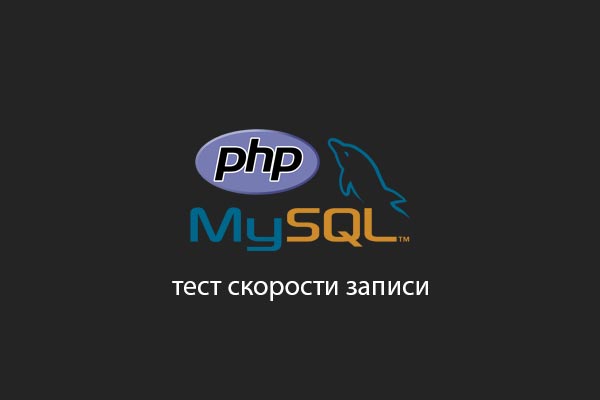
PHP: скрипт для теста скорости записи данных в MySQL
Напишем скрипт, для проверки скорости записи данных в mysql.
Этот скрипт-шаблон подключается к базе MySQL, подготавливает оператор вставки SQL с использованием расширения mysqli, а затем в цикле записывает указанное количества строк. При этом, измеряет время, затраченное на вставку всех строк, и в конце распечатывает результат.
Каждая строка прокомментирована, поэтому вы легко сможете конфигурировать скрипт под вашу базу и таблицу.
<?php
// Connect to the MySQL server
$link = mysqli_connect("hostname", "username", "password", "database_name");
// Check for connection errors
if (!$link) {
die("Error: Could not connect to the MySQL server. " . mysqli_connect_error());
}
// Prepare the SQL insert statement
$stmt = mysqli_prepare($link, "INSERT INTO table_name (column1, column2, column3) VALUES (?, ?, ?)");
// Bind the variables to the statement
mysqli_stmt_bind_param($stmt, "sss", $column1, $column2, $column3);
// Start the timer
$start_time = microtime(true);
// Number of rows to insert
$num_rows = 100000;
// Insert the rows
for ($i = 0; $i < $num_rows; $i++) {
// Assign values to the variables
$column1 = "value1";
$column2 = "value2";
$column3 = "value3";
// Execute the insert statement
mysqli_stmt_execute($stmt);
}
// Calculate the total time
$total_time = microtime(true) - $start_time;
// Print the results
echo "Inserted $num_rows rows in $total_time seconds.";
// Close the statement and connection
mysqli_stmt_close($stmt);
mysqli_close($link);