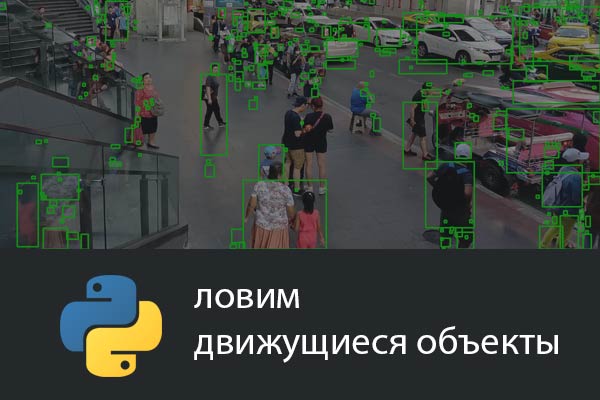
Python: скрипт для распознавания движущихся объектов на видео
Напишем скрипт на Python, который будет распознавать движущиеся объекты на видео и обводить их рамкой.
Для этого будем использовать библиотеку cv2. Импортируем ее в верху файла, и указываем в методе VideoCapture путь к вашему видеофайлу с расширением.
import cv2
capture = cv2.VideoCapture('video.mp4')
После чего инициализируем субстрактор фона и запускаем цикл с основной логикой.
bgSubtractor = cv2.createBackgroundSubtractorMOG2()
Весь код для захвата объектов с комментариями
Обратите внимание, что алгоритм захватывает не только людей и автомобили. Но в том числе блики, тени и отражения.
import cv2
# Set up the video capture
capture = cv2.VideoCapture('video.mp4')
# Set up the background subtractor
bgSubtractor = cv2.createBackgroundSubtractorMOG2()
while True:
# Read a frame from the video
ret, frame = capture.read()
# Check if the video has ended
if not ret:
break
# Use the background subtractor to detect moving objects
fgMask = bgSubtractor.apply(frame)
# Use morphological operations to remove noise from the foreground mask
kernel = cv2.getStructuringElement(cv2.MORPH_ELLIPSE, (5,5))
fgMask = cv2.morphologyEx(fgMask, cv2.MORPH_OPEN, kernel)
# Find contours in the foreground mask
contours, _ = cv2.findContours(fgMask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Iterate over the contours and draw rectangles around the moving objects
for c in contours:
x,y,w,h = cv2.boundingRect(c)
cv2.rectangle(frame, (x,y), (x+w, y+h), (0,255,0), 2)
# Display the frame with the moving objects highlighted
cv2.imshow('Frame', frame)
# Check if the user has pressed the 'q' key to quit
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the video capture and destroy the window
capture.release()
cv2.destroyAllWindows()